How To Create A Sliding Image Scrolling Preloader With JavaScript
Image preloaders are a common sight on many websites. They can be used to improve the user experience by providing feedback on the loading status of images. In this tutorial, we will learn how to create a sliding image scrolling preloader with JavaScript.
We will start by creating a basic HTML structure for our preloader. This will include a container element and an image element. We will then add some CSS to style the container and image. Next, we’ll create a function that will update the position of the image as it is scrolled. Finally, we’ll add an event listener that will call our function when the page is scrolled.
The HTML for our preloader will look like this:

The CSS for our preloader will look like this:
#container {
width: 100%;
height: 100%;
overflow: hidden;
}
#container img {
width: 100%; /* image must be same size as container */ /* set position so top of image is visible */ object-fit: cover; /* hide extra space below image */ } }
And the JavaScript will look like this:
// get container element var container = document.getElementById(‘container’); // get image element var image = document.querySelector(‘#container
inserting image in jupyter notebook
In order to insert an image into a Jupyter notebook, you will need to use the  command. The exclamation mark tells the notebook that you are about to enter a system command, and the parentheses indicate that you are passing in an argument (in this case, the URL of the image you want to insert).
For example, if you wanted to insert the image http://example.com/image.jpg into your notebook, you would use the following command:

If you want to align the image to the left or right of your text, you can use the following syntax:
{: .align-left} {: .align-right}
You can also control the width and height of your images by using the following syntax:
{: width=100px height=100px}
image downloader from url
When it comes to creating a sliding image scrolling preloader with JavaScript, one of the most important things to consider is the image downloader from URL. This tool will allow you to download images from a given URL so that you can use them in your preloader.
There are a few different ways that you can go about downloading images from a URL. One way is to use a third-party tool such as wget or cURL. Another way is to use a script that you can run from the command line.
whichever method you choose, make sure that you download the image to a location that is accessible to your preloader script. Once the image is downloaded, you can then reference it in your preloader code.
If you decide to use a third-party tool, there are a few things to keep in mind. First, make sure that the tool supports the URL scheme that you are using. Second, make sure that you set the output file name to something that makes sense. Finally, make sure that you understand how the tool works so that you can properly configure it.
If you decide to use a script, there are also a few things to keep in mind. First, make sure that the script supports the URL scheme that you are using. Second, make sure that you set the output file name to something that makes sense. Finally, make sure that you understand how the script works so that you can properly configure it.
Define what a preloader is and how it’s used.
A preloader is a component that helps improve the loading time of web pages and applications. It allows the user to see the content that is being loaded in the background, so they know that something is happening even if it’s taking a while. This can be especially helpful when loading large files or many files at once. Preloaders are often used in games and other interactive applications to give the user a better experience.
Discuss the benefits of using a preloader.
Preloading assets is a common practice in game development and web design. The purpose of preloading is to load assets before they are needed, so that they can be used immediately when required. This eliminates any delay caused by loading the asset on demand.
There are several benefits to using a preloader:
1. Reduces lag and stuttering – By loading assets ahead of time, you can avoid having to wait for them to load when you need them. This is especially important in games, where even a short delay can ruin the experience.
2. Makes better use of bandwidth – If you’re loading assets on demand, there’s a good chance that some of them will never be used. Preloading ensures that all assets are loaded, so none of your bandwidth goes to waste.
3. Allows for more control over the order in which assets are loaded – With preloading, you can decide exactly what order your assets should be loaded in. This can be useful if certain assets depend on others (eg: loading level data before terrain geometry).
Introduce the JavaScript code needed to create a preloader.
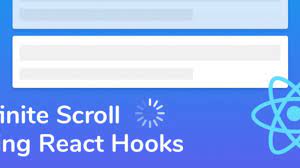
JavaScript code is needed to create a preloader for your web page. This code will make sure that all of the content on your page is loaded before displaying it to the user. This will help improve the overall experience for the user, as they will not have to wait for each element to load individually.
To implement this code, you will need to add it to the head of your HTML document. You can do this by adding the following line:
Once you have added this line, you will need to create a new file called “preloader.js” and save it in the same directory as your HTML file. The contents of this file should be as follows:
window.onload = function() {
document.getElementById(‘page-container’).style.display = ‘block’;
}
Show how to implement the preloader code into a website.
Preloaders are a great way to improve the user experience on your website. They can help reduce the perceived load time of your site, and can also be used to provide visual feedback to the user while assets are being loaded in the background.
In this tutorial, we’ll show you how to implement a preloader on your website using JavaScript. We’ll also cover how to automatically trigger the preloader when necessary, and how to hide it when all assets have been successfully loaded.
Test the preloader to ensure it works properly.
1. Click on the “Settings” icon in the upper right corner of the page.
2. Select “Advanced settings” from the drop-down menu.
3. Scroll down to the “Preloader” section and click on the “Test preloader” button.
4. The preloader will now start loading resources and you should see a progress bar indicating its status.
5. Once the preloader is finished, you can close this window and continue using the site as normal.
color overlay image css
When creating a preloader for your website, you may want to consider using a color overlay image. This can provide a more visually appealing preloading experience for your users.
To create a color overlay image preloader, you will need to use CSS to overlay a color onto your image. You can do this by using the CSS property “background-color”. For example, let’s say you have an image that is 400px by 400px. You would want to add the following CSS to your image:
background-color: #000000;
This would add a black background color to your image. You can adjust the hex code to change the color of the background.
In addition to setting a background color, you may also want to set a background image. This can be done by using the CSS property “background-image”. For example, let’s say you have an image of a cityscape that you want to use as your background image. You would add the following CSS to your element:
background-image: url(“cityscape.jpg”);
You can also use CSS3 properties to create an animation for your color overlay image preloader. For example, you could use the “animation” property to create a fading effect:
animation: fadeIn 2s;
This would create a two second animation where the element fades in from transparent to solid.