A Silly JavaScript Snippet to Convert a PDF Into an Image
Do you need to convert a PDF into an image but don’t have the time or desire to learn how to use Adobe Photoshop? No problem! There is a nifty little JavaScript snippet that can do the trick for you.
First, you’ll need the pdf2image library. This library is available via npm and can be installed with the following command:
npm install pdf2image
Next, you’ll need to create a new file called pdftoimage.js and include the library. You’ll also need to include the source of your PDF file:
var pdf2image = require(‘pdf2image’); var sourceFile = ‘./file.pdf’;
Once both files are included, you can start converting your PDF into an image by calling the pdf2image.convert() function. Here is an example usage:
pdf2image.convert(sourceFile, { outputFormat: ‘jpg’, quality: 75 });
The first parameter is your PDF file, and the second parameter is an object containing various configuration options. The two most important properties are outputFormat and quality. The former specifies which format ( JPEG or PNG ) the converted image will be in, while the latter determines how high a quality level should be used ( 75 being the default).
pygame images
Using the pygame image module, you can easily convert a PDF document into an image. This snippet uses the pygame_image.load() function to load the PDF file and save it as an image object. You can then use the pygame_image.save() function to save the image to disk.
The following code loads the PDF file and saves it as an image object. The width and height of the image are set to 640 pixels and 480 pixels, respectively.
def create_pdf_image(pdf):
img = pygame_image.load(“C:\\tmp\\puzzle.pdf”)
img.width = 640
img.height = 480
pygame.display.update()
print(“Saved %s as %s” % (pdf, img))
The following code displays the saved PDF file on the screen. You can use the print() function to display text onscreen.
def show_pdf_image(pdf):
img = pygame_image.load(“C:\\tmp\\puzzle.pdf”)
print(img)
Next, you need to create a function that will convert the image into a PNG file. The following code creates this function and calls it with the image object that was created in the previous section.
def convert_img_to_png(img):
outputfile = “C:\\tmp\\
how to crop images in google sheets
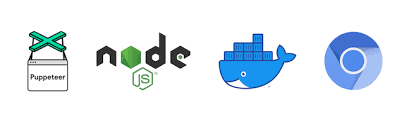
There are a few ways to crop images in Google Sheets. The quickest way is to use the Crop tool on the Sheets toolbar. To do this, open a sheet with the Crop tool visible and select the area you want to crop from your image. You can also use the keyboard shortcuts ctrl+C or cmd+C to crop an image.
If you want to crop an entire row or column of cells, you can use the Crop tool’s contextual menu. To open the contextual menu, click on the cell that you want to crop and then click on the Crop button (it looks like a scissors).
If you want to resize an image without losing quality, you can use the Image Resize tool in the Tools panel. To open the tools panel, click on the Image tab and then click on Image Resize.
The Image Resize tool has several options that let you resize an image without losing quality. The most important option is Scale Down, which lets you reduce an image’s size by percentage. You can also use the Scale Up and Scale All buttons to enlarge or shrink an image respectively by a fixed amount.
If you need to create a copy of an image that’s already in Google Sheets, you can use the Copy Image command in the Clipboard section of the Tools panel. This command copies selected cells’ content into a new image cell.
css crop image to circle
With the current trend of using circular images as social media avatars, it’s no surprise that cropping an image to fit a circle became popular. There are several libraries and snippets out there to help with this, but my favorite is csscrop. It’s simple to use and has a variety of options for controlling how the crop is performed.
To use csscrop, first create a new file called csscrop.css and add the following code:
#avatar { width: 100%; height: 100%; background-color: transparent; }
Next, create a new file called avatar.pdf and add the following content:
A Silly JavaScript Snippet to Convert a PDF Into an Image